So, life has it that I’m writing a wrapper for Google Charts. Life also has it that I get this blog. My life rocks - What more can I say?
Actually, I will say that the API as it stands at time of publishing is below.
Perhaps the first aspect that I should point out is that there are three main elements:
- The line charts. There are two types of line chart: the basic line chart and the timeline chart. The basic line chart is just that: a line chart. It has a X axis and a Y axis. They have labels. There are data series. The timeline chart is the line chart but is a special case made just for data where the X axis is in units of time.
- The table chart. This is a lot simpler than the line charts. It just displays data in the form of a table. The most complex part here is the initial sorting of the table. I’ll get to that in just a minute.
- Last and most likely least, the peak analysis. This is really just a utility function to help with peak analysis - at the moment it’s used in one place, and I don’t expect it to go much further. But I will discuss it anyways.
Basic Line Charts
They aren’t hard. Really. I promise.
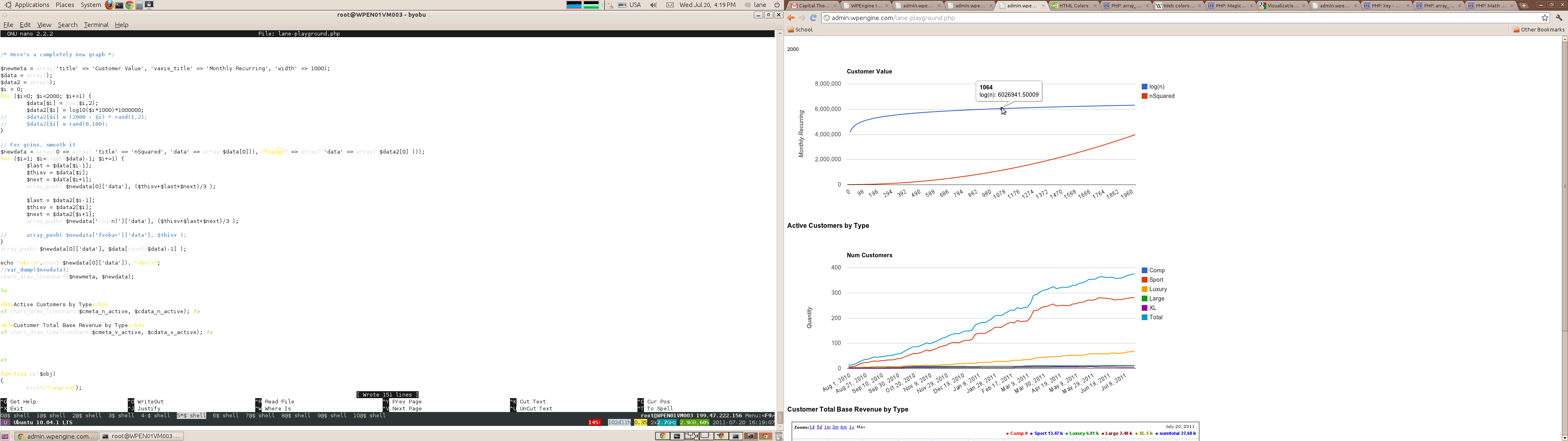
The red line is n squared, where n is a number that loops from 0 through 2000 (and is represented on the X-axis), and the blue line is the log of that number.
So you can see the basic properties of this graph: two data series, labelled X & Y axes, a legend, a title (“Customer Value”) and a vertical axis title (“Monthly Recurring”).
The above graph was created with a single function call (this ain’t class based). Two arguments were passed to that function: The meta array and the data array.
The meta array takes the form of a map from meta keys to meta values. A list of possible meta tags and their values are:
title => [string] | Sets the title of the graph. |
haxis_title => [string] | Sets the title of the X-axis. |
vaxis_title => [string] | Sets the title of the Y-axis. |
width => [int] | Sets the width of the graph, in pixels. |
height => [int] | Sets the height of the graph, in pixels. |
compute_sum => [true/false] | Computes and graphs the sum of all data series's. |
The data is also an associative array. However, this maps series names to the series itself (which is, surprisingly, another associative array). Note that the order that the series’s are presented are important for the timeline graph, but not for this graph. Yet.
The array that represents the series contains both the metadata about the series and the data itself. The only meta tag allowed here is ‘title’: You can use it to specify the title of the series.
Here’s the PHP code snippet that generated the above graph:
$newmeta = array('title' => 'Customer Value', 'vaxis_title' => 'Monthly Recurring', 'width' => 1000); $data = array(); $data2 = array(); $i = 0; for ($i=0; $i<2000; $i+=1) { $data[$i] = pow($i,2); $data2[$i] = log10($i*1000)*1000000; } $newdata = array(0 => array( 'title' => 'nSquared', 'data' => array($data)), "log(n)" => array( 'data' => array( $data2 ))); chart_draw_linechart($newmeta, $newdata);
Note how this code demonstrates both forms of specifying titles: Using the title as the key, and putting the title inside the series metadata.
Timeline Charts
These are EXACTLY THE SAME, except easier: they don’t have as many meta tags.
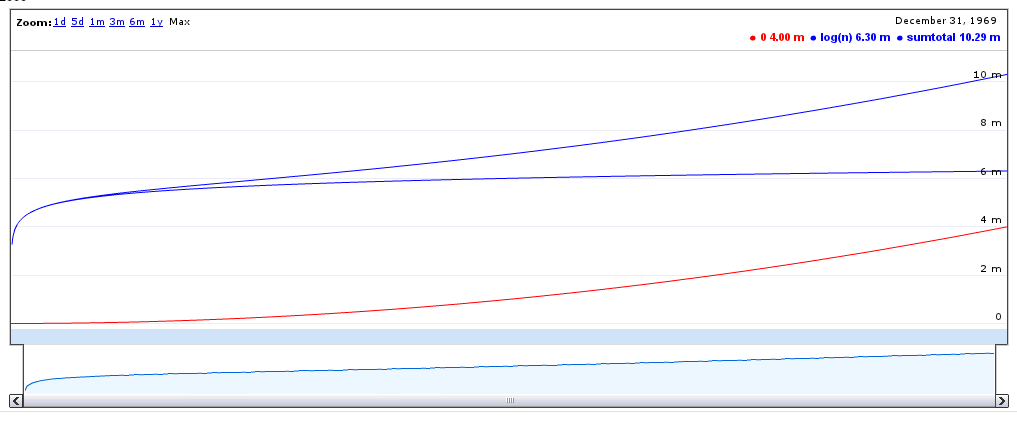
This particular chart has the sum total feature enabled, which is optional and works for the standard line chart as well. The sum total feature enables the window view at the bottom (the light blue thing). That line is generated from the log of the total. It is not present if totaling is turned off. This chart also has the neat feature that you can zoom in and out and scroll around and stuff. It’s implemented in Flash, while the others are implemented in JavaScript.
The allowable meta keys for this graph are:
set_null_to_zero => [true/false] | If true, then missing values are set to zero, instead of interpolating. |
width => [int] | Sets the width of the graph, in pixels. |
height => [int] | Sets the height of the graph, in pixels. |
compute_sum => [true/false] | Computes and graphs the sum of all data series's. |
And the code snippet generated this graph in action is:
$newmeta = array('title' => 'Customer Value', 'vaxis_title' => 'Monthly Recurring', 'width' => 1000, 'compute_sum' => true); $data = array(); $data2 = array(); $i = 0; for ($i=0; $i<2000; $i+=1) { $data[$i] = pow($i,2); $data2[$i] = log10($i*1000)*1000000; } $newdata = array(0 => array( 'title' => 'nSquared', 'data' => array($data)), "log(n)" => array( 'data' => array( $data2 ))); chart_draw_linechart($newmeta, $newdata);
Tables
I won’t include a screenshot. We all know what tables are like. If you must see a sample, look at the example on http://code.google.com/apis/chart/interactive/docs/gallery/table.html. (Or look at the backend analysis. Those are tables).
This one, unlike the others, takes two associative arrays which represent columns and data, respectively. Note how there is no place to put frivolous metadata: It’s kind of handy that way.
The columns array takes in column identifiers as keys and column arrays as values. The column arrays in turn contain two things: The name (identified by the ‘name’ tag) of the column and the type of the column (the ‘type’ tag). The type value does take some special care, as it formats data according to the type, as specified in the table below:
money | Formats with a '$', rounds to the nearest dollar. |
smallmoney | Formats with a '$', rounds to the nearest cent. |
number | Formats integer with commas (uses number_format). |
secs | Appends 'secs' to the end, and round to the nearest second. |
monthtime | Formats as a month and year, e.g. 'May 2011' |
The data array is just an array of rows. Each row must be associative. The pieces of data that are actually drawn are specified by the key that you specified in the columns array: The two keys will match.
Here’s some sample code:
$columns = array('account_name' => array('name' => 'Account Name', 'type' => 'string') ,'profit' => array('name'=>'Profit', 'type' => 'money') ,'revenue' => array('name'=>'Revenue', 'type' => 'money') ,'expenses' => array('name'=>'Cost', 'type' => 'money') ); $chartdata = array(); foreach ($data as $site) { if ($site['profit'] < PROFIT_LOSS_THRESHOLD) { array_push($chartdata, $site); } } chart_draw_table( $columns, $chartdata );
Happy Coding!